This article will show you how to integrate @observablehq/plot with Angular.
Observable Plot is a free, open-source, JavaScript library for visualizing tabular data, focused on accelerating exploratory data analysis
Use case
I want to use plot to draw a “hello world” banner in Angular application
Install required dependencies
npm install @observablehq/plot
Create Plot object
Once you have plot installed, import it to your component file
import * as Plot from '@observablehq/plot';
Define a component template to render plot object, in this example, I simply defined a div tag with innerHtml attribute to hold a chart object, in the component ngOnInit method, create a plot object output as html object.
@Component({
selector: 'my-app',
standalone: true,
imports: [CommonModule],
template: `
<h1>Hi Plot</h1>
<div [innerHtml]="chart"></div>
`,
})
export class App implements OnInit {
chart: any;
ngOnInit(): void {
this.chart = Plot.plot({
marks: [
Plot.frame(),
Plot.text(['Hello, world!'], { frameAnchor: 'middle' }),
],
}).outerHTML;
}
}
Check UI
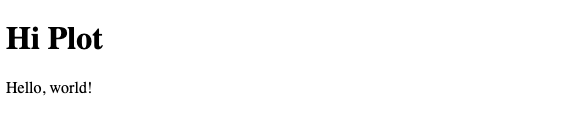
Full example source code can be found at Plot Example